Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial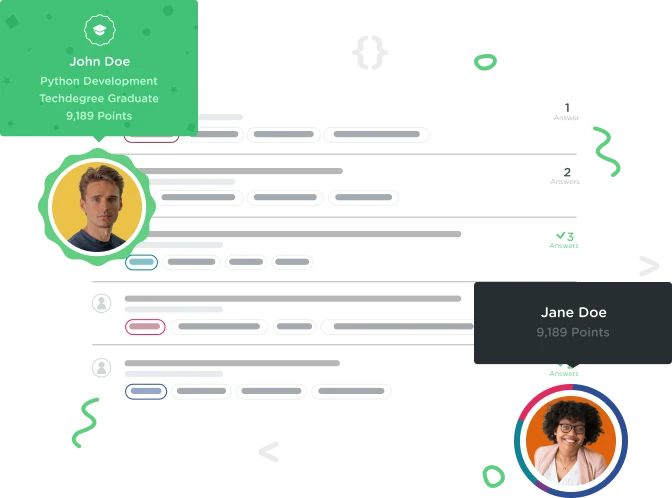
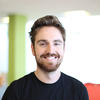
Kristian Woods
23,414 PointsWhy when I throw a new Error in a try/catch, the rest of my script stops executing?
I have created a basic es6 class. I'm using a try/catch
block throughout the class. However, in my catch block, when I write:
try {
// Do something
} catch (error) {
throw new Error('No string to perform lower method on');
}
The error is thrown, however, the rest of my script stops running. However, if I replace the throw new Error
with console.error()
- my script continues just fine.
Here is my class in full:
class StringFormatter {
constructor(props) {
try {
// Check if the value passed to props.string is valid
if (
props.string === null ||
props.string === undefined ||
props.string.length <= 0 ||
typeof props.string !== "string"
) {
throw new Error(`can't find string - value provided: "${props.string}"`)
}
} catch (error) {
console.error(error);
}
}
get stringVal() {
console.log(this._string);
return this._string;
}
set stringVal(val) {
this._string = val;
}
lower() {
// try to run lower() method, if not, throw error
try {
this.stringVal = this._string.toLowerCase();
return this;
} catch (error) {
throw new Error('No string to perform lower method on');
}
}
replaceSpaces(val) {
// try to run replaceSpaces() method, if not, throw error
try {
this.stringVal = this._string.replace(/\s+/g, val);
return this;
} catch (error) {
throw new Error('No string to perform replaceSpaces method on');
}
}
}
// initiate StringFormatter class and provide an empty string for error testing
let myString = new StringFormatter({
string: ''
});
// perform methods on new instance of class
myString.lower().replaceSpaces("*").stringVal;
// execute alert() - this should always run, even if the class object fails to execute methods.
alert("continues with the rest of script");
I'm trying to set it up, so that if the myString
instance fails to run the methods, the rest of my script will continue to run. However, right now, that isn't the case. The alert()
never runs.
1 Answer
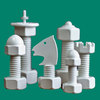
Steven Parker
231,141 PointsA "throw" that is not caught will always cause the program to end. And only code that is inside a "try" block can be caught.
So the "throw" shown here inside the "catch" block is not in a "try", does not get caught, and instead gets handled by the system which ends the program. It's almost as if there were no "try/catch" at all except that this code changes the original event for a different new one.
If your intention is for the code to continue running, then issuing a message instead of performing a "throw" is the appropriate thing to do.