Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial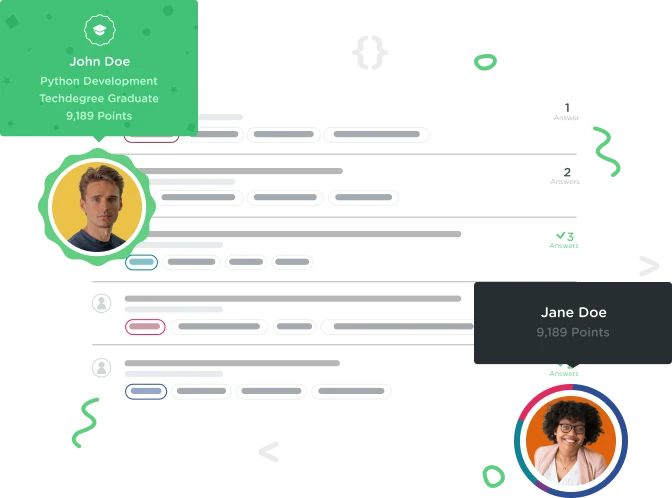

Kevin Gates
15,053 PointsWhy won't my DataBase Initializer work? (Visual Studio 2017)
Why won't my DataBase Initializer work? (Visual Studio 2017) Before I move it to the initializer class, it works. But starting with this lesson, my program won't load the comics in the Console Window, I don't get an error, and I never get to my breakpoint.
DatabaseInitializer.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Data.Entity;
using ComicBookGalleryModel.Models;
namespace ComicBookGalleryModel
{
internal class DatabaseInitializer
: DropCreateDatabaseIfModelChanges<Context>
{
protected void Seed(Context context)
{
var series1 = new Series() { Title = "The Amazing Spider-Man" };
var series2 = new Series() { Title = "The Invincible Iron Man" };
var artist1 = new Artist() { Name = "Stan Lee" };
var artist2 = new Artist() { Name = "Steve Ditko" };
var artist3 = new Artist() { Name = "Jack Kirby" };
var role1 = new Role() { Name = "Script" };
var role2 = new Role() { Name = "Pencils" };
var comicBook1 = new ComicBook() { Series = series1, IssueNumber = 1, PublishedOn = DateTime.Today };
comicBook1.AddArtist(artist1, role1);
comicBook1.AddArtist(artist2, role2);
var comicBook2 = new ComicBook() { Series = series1, IssueNumber = 2, PublishedOn = DateTime.Today };
comicBook2.AddArtist(artist1, role1);
comicBook2.AddArtist(artist2, role2);
var comicBook3 = new ComicBook() { Series = series2, IssueNumber = 1, PublishedOn = DateTime.Today };
comicBook3.AddArtist(artist1, role1);
comicBook3.AddArtist(artist3, role2);
context.ComicBooks.Add(comicBook1);
context.ComicBooks.Add(comicBook2);
context.ComicBooks.Add(comicBook3);
context.SaveChanges();
}
}
}
Context.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Data.Entity;
using ComicBookGalleryModel.Models;
using System.Data.Entity.ModelConfiguration.Conventions;
namespace ComicBookGalleryModel
{
public class Context : DbContext
{
public Context()
{
// Database.SetInitializer(new DropCreateDatabaseIfModelChanges<Context>());
Database.SetInitializer(new DatabaseInitializer());
}
public DbSet<ComicBook> ComicBooks { get; set; }
protected override void OnModelCreating(DbModelBuilder modelBuilder)
{
modelBuilder.Conventions.Remove<PluralizingTableNameConvention>();
//modelBuilder.Conventions.Remove<DecimalPropertyConvention>();
//modelBuilder.Conventions.Add(new DecimalPropertyConvention(5, 2));
modelBuilder.Entity<ComicBook>().Property(cb => cb.AverageRating).HasPrecision(5, 2);
}
}
}
Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using ComicBookGalleryModel.Models;
using System.Data.Entity;
namespace ComicBookGalleryModel
{
class Program
{
static void Main(string[] args)
{
using (var context = new Context())
{
var comicBooks = context.ComicBooks
.Include(cb => cb.Series)
.Include(cb => cb.Artists.Select(a => a.Artist))
.Include(cb => cb.Artists.Select(a => a.Role))
.ToList();
foreach (var comicBook in comicBooks)
{
var artistRoleNames = comicBook.Artists
.Select(a => $"{a.Artist.Name} - {a.Role.Name}").ToList();
var artistsRoleDisplayText = string.Join(", ", artistRoleNames);
Console.WriteLine(comicBook.DisplayText);
Console.WriteLine(artistsRoleDisplayText);
}
Console.ReadLine();
}
}
}
}
3 Answers

James Churchill
Treehouse TeacherKevin,
You're missing the override
keyword on your Seed()
method. Your method signature should look like this:
protected override void Seed(Context context)
{
}
Without the override
keyword, your Seed()
method is actually hiding the base class's Seed()
method. You should be able to verify this by compiling your project and looking at the build output in Visual Studio. You should see a warning that your Seed()
method is hiding the base class's method of the same name. The build warning will mention that you can add the new
keyword to suppress the warning, but that's not what you want to do.
When the Seed()
method is called at runtime, Entity Framework is likely calling it from the base type, so the base class's default implementation is what's getting executed. Once you add the override
keyword, your method implementation will be executed instead (i.e. you're "overriding" the base class's method).
I hope this helps!
Thanks ~James

Kevin Gates
15,053 PointsThis was dramatically helpful. Thank you, James Churchill .
Related but different topic: Visual Studio 2017 doesn't let you import databases now via a Wizard. You have to do this: https://docs.microsoft.com/en-us/ef/core/managing-schemas/scaffolding
With the VS 2019 coming soon, it may require a series update. I've been trying to learn and connect the dots of Entity Framework, WebAPI, Stored Procedures, and front end frameworks like Vue.
I'd love to at least hear a comparison video like you all sometimes do on Plan SQL, EF, Stored Procedures, etc.
Additionally, if you have any other classes like this one: https://teamtreehouse.com/library/php-user-authentication but for .NET stuff would be great. :)
You're a great teacher. Thanks again!
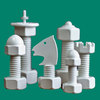
Steven Parker
231,269 PointsThe initializer code doesn't run until a change is made to the model. In the video, a test property is added to the "Artist" model (in Artist.cs, not shown here) to cause the code to run.
Did you do that (or make some other model change)?

Kevin Gates
15,053 PointsSteven Parker Yes, I added it, removed it, etc. I even deleted the database so it would create a new one -- no joy.
Kevin Gates
15,053 PointsKevin Gates
15,053 PointsJames Churchill or Steven Parker ?