Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial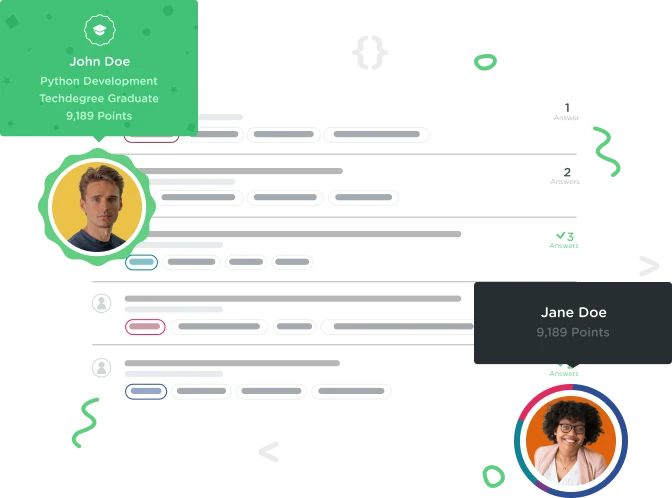

Chris M
592 PointsWorks in workspace but throws an error in testing challenge.
For some reason, this code works perfectly in the workspace. But when I use it in the task challenge it keeps giving me an error.
using System;
namespace Treehouse.CodeChallenges {
class Program {
static void Main() {
while (true) {
// parse the amount of times you want yay printed out
Start:
Console.Write("Enter the number of times to print \"Yay!\": ");
var ToRpeat = Console.ReadLine();
// make this all one massive while loop
// Catching entries that aren't numbers here
// while loop starts here
// Display Yay and then sutract 1 from var 'total'
try {
var total = int.Parse(ToRpeat);
while (true) {
if (total == 0) {
break;
}
if (total < 0) {
Console.WriteLine("You must enter a whole number.");
goto Start;
}
Console.WriteLine("Yay!");
total = total - 1;
}
} catch (FormatException) {
Console.WriteLine("You must enter a whole number.");
continue;
}
break;
}
}
}
}
10 Answers

anil rahman
7,786 PointsThis will do task 2
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
int num = 0;
try
{
num = Convert.ToInt32(Console.ReadLine());
}
catch(FormatException fe)
{
Console.Write("You must enter a whole number.");
}
int i = 0;
while(i < num) //counter is less than typed number
{
i++; //add 1 to the counter each time you loop
Console.Write("Yay!");
}
}
}
}

anil rahman
7,786 PointsThat is so complicated and long winded. Keep short and simple :)
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
int num = Convert.ToInt32(Console.ReadLine());
for(int i = 0; i < num; i++)
{
Console.Write("Yay!");
}
}
}
}

Chris M
592 PointsOne thing lead to another and it eventually turned into that beast , haha.
In any case, the program also has to catch exceptions for the test, for whatever reason and I haven't got to for loops yet.

anil rahman
7,786 PointsBut you seem fine with while loops, so this is a for loop converted to a while :)
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
int num = Convert.ToInt32(Console.ReadLine());
int i = 0;
while(i < num) //counter is less than typed number
{
i++; //add 1 to the counter each time you loop
Console.Write("Yay!");
}
}
}
}

Chris M
592 PointsYour right! Man, forgot how much I loved for loops.. Haven't touched programming in a while so I'm beyond rusty. i'll try this again, thanks.

anil rahman
7,786 PointsI don't see anything asking for exception checks so that would be extra stuff you can add yourself after :)

Chris M
592 PointsLooks like I'm still getting the same error.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
while (true)
{
Start: Console.Write("Enter the number of times to print \"Yay!\": ");
var ToRpeat = Console.ReadLine();
try {
var total = int.Parse(ToRpeat);
for (int i = 0; i < total; i++)
{
if (total == 0)
{
break;
}
Console.WriteLine("Yay!");
}
if (total < 0)
{
Console.WriteLine("You must enter a whole number.");
goto Start;
}
} catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
break;
}
}
}
}
I keep getting this error:
System.ArgumentNullException: Value cannot be null. Parameter name: String at System.Number.StringToNumber (System.String str, NumberStyles options, System.NumberBuffer& number, System.Globalization.NumberFormatInfo info, Boolean parseDecimal) [0x00054] in /builddir/build/BUILD/mono-4.2.3/external/referencesource/mscorlib/system/number.cs:1080 at System.Number.ParseInt32 (System.String s, NumberStyles style, System.Globalization.NumberFormatInfo info) [0x00014] in /builddir/build/BUILD/mono-4.2.3/external/referencesource/mscorlib/system/number.cs:751 at System.Int32.Parse (System.String s) [0x00000] in /builddir/build/BUILD/mono-4.2.3/external/referencesource/mscorlib/system/int32.cs:140 at Treehouse.CodeChallenges.Program.Main () <0x41b94830 + 0x00058> in :0 at MonoTester.Run () [0x00197] in MonoTester.cs:125 at MonoTester.Main (System.String[] args) [0x00013] in MonoTester.cs:28
Not sure what the deal is. Works fine in the workspace but as soon as I enter it into the test, stuff happens.

anil rahman
7,786 PointsWhy are you doing exception catching and number checking. The test doesn't ask you to do any of that. Also sometimes if you do extra things than what they ask for you can get the bummer error even if your code is correct. :)

Chris M
592 PointsWell the directions say "Add input validation to your program by printing “You must enter a whole number.” if the user enters a decimal or something that isn’t a number. Hint: Catch the FormatException."
So I figured I had to. I'm on task 2 of 3.

anil rahman
7,786 PointsThat's why i gave you the code to pass the test :)

anil rahman
7,786 PointsOops my bad i didn't see that -_-

Chris M
592 PointsAll good! Just wish I knew what is going on. I want to just skip it but if it's a bug then I feel like people should know.

anil rahman
7,786 PointsThis will do task 2
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
int num = 0;
try
{
num = Convert.ToInt32(Console.ReadLine());
}
catch(FormatException fe)
{
Console.Write("You must enter a whole number.");
}
int i = 0;
while(i < num) //counter is less than typed number
{
i++; //add 1 to the counter each time you loop
Console.Write("Yay!");
}
}
}
}

anil rahman
7,786 PointsThis will do task 2
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
int num = 0;
try
{
num = Convert.ToInt32(Console.ReadLine());
}
catch(FormatException fe)
{
Console.Write("You must enter a whole number.");
}
int i = 0;
while(i < num) //counter is less than typed number
{
i++; //add 1 to the counter each time you loop
Console.Write("Yay!");
}
}
}
}

Lomaloma Pepine
4,927 PointsThis also will help..
Cheers
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
while(true)
{
try
{
Console.Write("Enter the number of times to print \"Yay!\": ");
int input = Convert.ToInt32(Console.ReadLine());
for(int i = 0; i < input; i++)
{
Console.Write("Yay!");
}
break;
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
}
}
}
}

Simon Coates
28,694 Pointsseems to get through with:
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
int loopsNumber;
try {
loopsNumber = int.Parse(Console.ReadLine());
if(loopsNumber >= 0){
while(loopsNumber > 0){
Console.WriteLine("Yay");
loopsNumber--;
}
} else {
Console.WriteLine("You must enter a positive number.");
}
} catch(FormatException){
Console.WriteLine("You must enter a whole number.");
}
}
}
}
I think what is happening is that it's running it, but since your code doesn't quite behave as it should, you're seeing an unexpected error in the test code. Without knowing about how the tests are performed, the error seems inexplicable. (I also started out looping until a valid input was entered, and i guess the test code didn't know to keep providing values)
nb: i appreciate that y'all have probably moved on or had psychotic breaks, so this is mostly for future reference.
Chris M
592 PointsChris M
592 PointsWell I finished task 2 and 3.
Thanks for the help, still not sure why it hated my code so much :(