Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial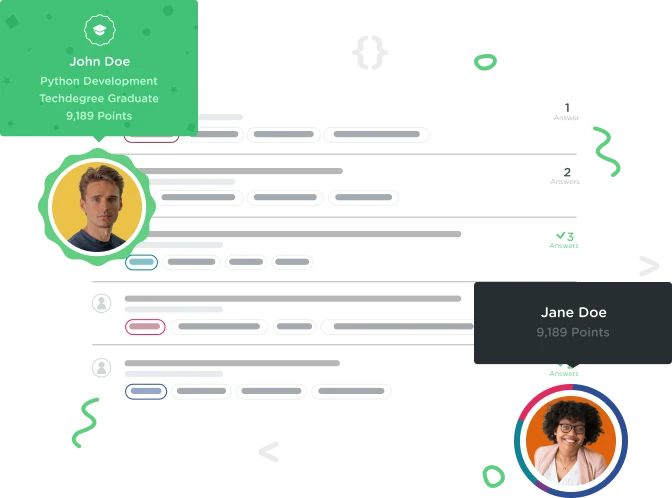

shirshah sahel
10,035 PointsWrite a program that translates a letter used in a telephone number into an equivalent numeric digit.
Topics
Branching
switch statement
Description
Write a program that translates a letter used in a telephone number into an equivalent numeric digit. The program asks the user to enter a letter, translates the letter into the corresponding digit and displays it. The user may enter its selection either as an upper or lower case letter.
The program translates the letter into a digit according to the table below:
Letters Digit
a b c 2
d e f 3
g h i 4
j k l 5
m n o 6
p q r s 7
t u v 8
w x y z 9
Testing
Input Test 1
Enter a letter: p
Output Test 1
Letter: p
Digit: 7
Input Test 2
Enter a letter: P
Output Test 1
Letter: P
Digit: 7
Sample Code 1
/*
The code below determines the character entered by user.
*/
/*
C++ code:
*/
char letter;
cout << “Enter a single alphabet:” << endl;
cin >> letter;
/*
Java code:
*/
/*
The code snippet below does the following:
It asks the user to enter a single alphabetical character
It saves the entered character in a string named in.
It extracts the first character from the String in.
It saves the extracted character in a char variable named letter.
*/
String in;
char letter;
int digit;
in = JOptionPane.showInputDialog(“Enter a single alphabet”);
letter = in.charAt(0);
Sample Code 2
/*
The code snippet below attempts to determine the digit corresponding to the letter.
Only a partially written Switch statement is shown. Add the additional cases.
*/
switch (letter)
{
case ‘a’:
case ‘b’:
case ‘c’:
case ‘A’:
case ‘B’:
case ‘C’:
digit = 2;
break;
case ‘d’:
case ‘e’:
case ‘f’:
case ‘D’:
case ‘E’:
case ‘F’:
digit = 3;
break;
}
I need the answer of this question in c++ language by using switch statement.
so far i have done this much.
```#include <iostream>
using namespace std;
in = JOptionPane.showInputDialog(ÒEnter a single alphabetÓ); letter = in.charAt(0);
int main() {
string in;
char letter;
int digit;
cout<< "Enter a single alphapet:"<<endl;
cin>> letter;
switch (letter)
{
case ‘a’:
case ‘b’:
case ‘c’:
case ‘A’:
case ‘B’:
case ‘C’:
digit = 2;
break;
case ‘d’:
case ‘e’:
case ‘f’:
case ‘D’:
case ‘E’:
case ‘F’:
digit = 3;
break;
}
return 0;
}
2 Answers
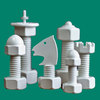
Steven Parker
231,110 PointsI should point out that you have posted this in a topic area intended for the "C#" language. But I happen to also be familiar with C++.
You seem to have a good start there, but you clearly have several more digits to implement. The process will be very similar to the digits you have done already. Did you have a question at this point?
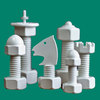
Steven Parker
231,110 PointsJava and C++ are two different languages. Mixing them will probably not produce a workable program.
And that said, they do have some similar constructs, so there may be some parts that look very similar, yet there may be some subtle differences in syntax.
But the code you show here is a good start, it looks like you mainly just need to implement the rest of the digits the same way as you have done for the first 2.

shirshah sahel
10,035 PointsThanks Paker, still not very clear on the answer. there was not any category to select C++ so I choose c# language. My main thing is how to use java code in C++ switch statement.
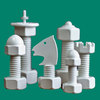
Steven Parker
231,110 PointsI added a comment to my answer. Also, FYI, there are courses here for the Java language (but not for C++).
Steven Parker
231,110 PointsSteven Parker
231,110 PointsWhen posting code, use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.