Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial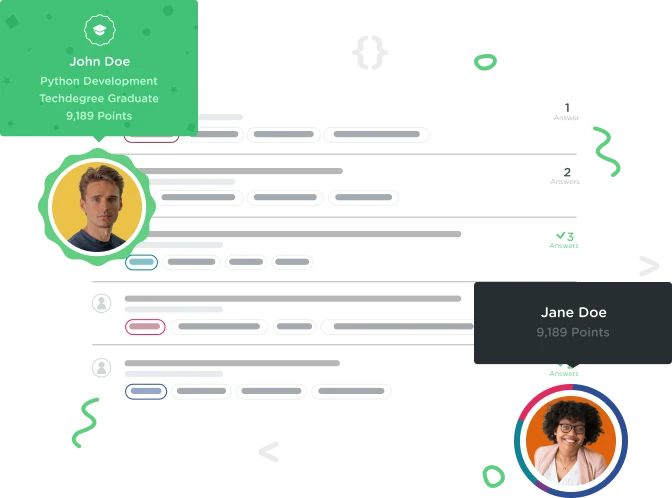

Richard Kerr
Courses Plus Student 946 PointsWrote C# program to get number of times to print "Yay!". Program seems to compile but not passing test. No error message
Don't know why this code is not passing the test. There are no compiler error messages. Program uses a loop to ensure valid data is entered. Code does not seem to satisfy the test. Why?
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
int timesToPrint = 0;
while(true)
{
var x = Console.ReadLine();
try
{
timesToPrint = int.Parse(x);
if(timesToPrint < 0)
throw new FormatException("Negative value entered.");
// Print Yay!
while(timesToPrint > 0)
{
Console.WriteLine("Yay!");
timesToPrint--;
}
break;
}
catch(FormatException e)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
catch(Exception ex)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
}
}
}
}
2 Answers
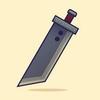
Allan Clark
10,810 PointsThe code does look good and like it will work, the TreeHouse platform for checking the answers may be having issues with going through the try-catch/Exception part of your code as input validation using exceptions is not part of the challenge objectives. Try something like this:
Console.Write("Enter the number of times to print \"Yay!\": ");
var countString = Console.ReadLine();
int count;
Int32.TryParse(countString, out count);
while(count > 0) {
Console.Write("Yay!");
count--;
}

Richard Kerr
Courses Plus Student 946 PointsThanks! Your suggestion works!
Richard Kerr
Courses Plus Student 946 PointsRichard Kerr
Courses Plus Student 946 PointsCompiles and executes as expected in VisualStudio 2015