Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial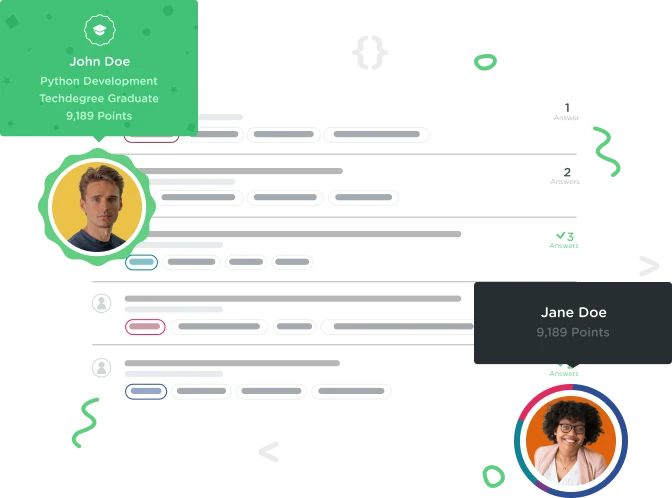
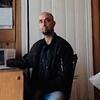
Louis Sankey
22,595 PointsTrouble with model view presenter in code challenge
I have the MainActivity implementing MainActivityView:
public class MainActivity extends AppCompatActivity implements MainActivityView{
TextView counter;
Button button;
MainActivityPresenter presenter;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
presenter = new MainActivityPresenter(this);
counter = (TextView) findViewById(R.id.textView);
button = (Button) findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
int count = Integer.parseInt(counter.getText().toString());
presenter.updateTextView(Integer.toString(count + 1));
}
});
}
@Override
public void changeTextViewText(String text){
counter.setText(text);
}
}
I don't know if I need one or two methods in my interface:
public interface MainActivityView {
void changeTextViewText(String text);
}
Likewise, I don't know if I need one or two methods in my presenter:
public class MainActivityPresenter {
MainActivityView view;
MainActivityPresenter(MainActivityView view){
this.view = view;
}
public void updateTextView(String text){
view.changeTextViewText(text);
}
}
The hint is saying I need to create a constructor for my presenter, but I think I already have one. Creating an interface method to handle the button click (or for incrementing the counter) is throwing me off. This is my first time learning MVP.
1 Answer

Boban Talevski
24,793 PointsYou need one method in the presenter and one method in the view. Think of them as action and reaction. The action method is specified in the presenter, the reaction method is specified in the view.
Though Ben says that methods in the view interface are for every action we can take on our view, it's still an action we take as a reaction to what the user did.
So, I have the "action" in the presenter named counterButtonClicked, and the "reaction" in the view named increaseCounterByOne. Meaning, the user clicks the button, that's the user action, and our app's (re)action is to increase the counter by one.
MainActivity.java
public class MainActivity extends AppCompatActivity implements MainActivityView {
TextView counter;
Button button;
MainActivityPresenter presenter;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
presenter = new MainActivityPresenter(this);
counter = (TextView) findViewById(R.id.textView);
button = (Button) findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
presenter.counterButtonClicked();
}
});
}
@Override
public void increaseCounterByOne() {
int count = Integer.parseInt(counter.getText().toString());
counter.setText(Integer.toString(count + 1));
}
}
MainActivityView.java
public interface MainActivityView {
public void increaseCounterByOne();
}
MainActivityPresenter.java
public class MainActivityPresenter {
MainActivityView view;
public MainActivityPresenter(MainActivityView view) {
this.view = view;
}
public void counterButtonClicked() {
view.increaseCounterByOne();
}
}
You have probably solved your particular problem by now, but aside from the possible problem with the public keyword before the constructor, there are some other things which need fixing.
You need the presenter to take care of the actual onClick method, so the code there should be replaced with the appropriate presenter "action" method (presenter.counterButtonClicked() in my case), which will in turn call the appropriate view "reaction" method (view.increaseCounterByOne() in my case).
And there's no need to pass arguments around in these methods because everything you need should be available to you already, as we are accessing the current value from the counter TextView and just increasing it.
Michael Nock
Android Development Techdegree Graduate 16,018 PointsMichael Nock
Android Development Techdegree Graduate 16,018 PointsI believe it's because they're expecting the 'public' keyword in front of your constructor. I just tested it with/without and without the public keyword it gave me the same message you got about creating a constructor.